|
| 1 | +3286\. Find a Safe Walk Through a Grid |
| 2 | + |
| 3 | +Medium |
| 4 | + |
| 5 | +You are given an `m x n` binary matrix `grid` and an integer `health`. |
| 6 | + |
| 7 | +You start on the upper-left corner `(0, 0)` and would like to get to the lower-right corner `(m - 1, n - 1)`. |
| 8 | + |
| 9 | +You can move up, down, left, or right from one cell to another adjacent cell as long as your health _remains_ **positive**. |
| 10 | + |
| 11 | +Cells `(i, j)` with `grid[i][j] = 1` are considered **unsafe** and reduce your health by 1. |
| 12 | + |
| 13 | +Return `true` if you can reach the final cell with a health value of 1 or more, and `false` otherwise. |
| 14 | + |
| 15 | +**Example 1:** |
| 16 | + |
| 17 | +**Input:** grid = [[0,1,0,0,0],[0,1,0,1,0],[0,0,0,1,0]], health = 1 |
| 18 | + |
| 19 | +**Output:** true |
| 20 | + |
| 21 | +**Explanation:** |
| 22 | + |
| 23 | +The final cell can be reached safely by walking along the gray cells below. |
| 24 | + |
| 25 | +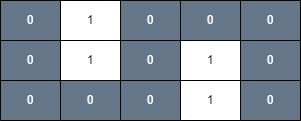 |
| 26 | + |
| 27 | +**Example 2:** |
| 28 | + |
| 29 | +**Input:** grid = [[0,1,1,0,0,0],[1,0,1,0,0,0],[0,1,1,1,0,1],[0,0,1,0,1,0]], health = 3 |
| 30 | + |
| 31 | +**Output:** false |
| 32 | + |
| 33 | +**Explanation:** |
| 34 | + |
| 35 | +A minimum of 4 health points is needed to reach the final cell safely. |
| 36 | + |
| 37 | +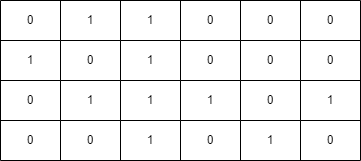 |
| 38 | + |
| 39 | +**Example 3:** |
| 40 | + |
| 41 | +**Input:** grid = [[1,1,1],[1,0,1],[1,1,1]], health = 5 |
| 42 | + |
| 43 | +**Output:** true |
| 44 | + |
| 45 | +**Explanation:** |
| 46 | + |
| 47 | +The final cell can be reached safely by walking along the gray cells below. |
| 48 | + |
| 49 | +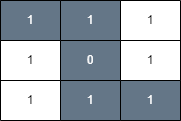 |
| 50 | + |
| 51 | +Any path that does not go through the cell `(1, 1)` is unsafe since your health will drop to 0 when reaching the final cell. |
| 52 | + |
| 53 | +**Constraints:** |
| 54 | + |
| 55 | +* `m == grid.length` |
| 56 | +* `n == grid[i].length` |
| 57 | +* `1 <= m, n <= 50` |
| 58 | +* `2 <= m * n` |
| 59 | +* `1 <= health <= m + n` |
| 60 | +* `grid[i][j]` is either 0 or 1. |
0 commit comments