|
| 1 | +# Array Questions |
| 2 | + |
| 3 | +[](https://github.com/ComputeNepal/learn-c-programming "Go to GitHub repo") |
| 4 | +[](https://github.com/ComputeNepal/learn-c-programming) |
| 5 | +[](https://github.com/ComputeNepal/learn-c-programming) |
| 6 | + |
| 7 | +[](#license) |
| 8 | +[](https://github.com/ComputeNepal/learn-c-programming/issues) |
| 9 | + |
| 10 | +[](/CONTRIBUTING.md "Go to contributions doc") |
| 11 | + |
| 12 | +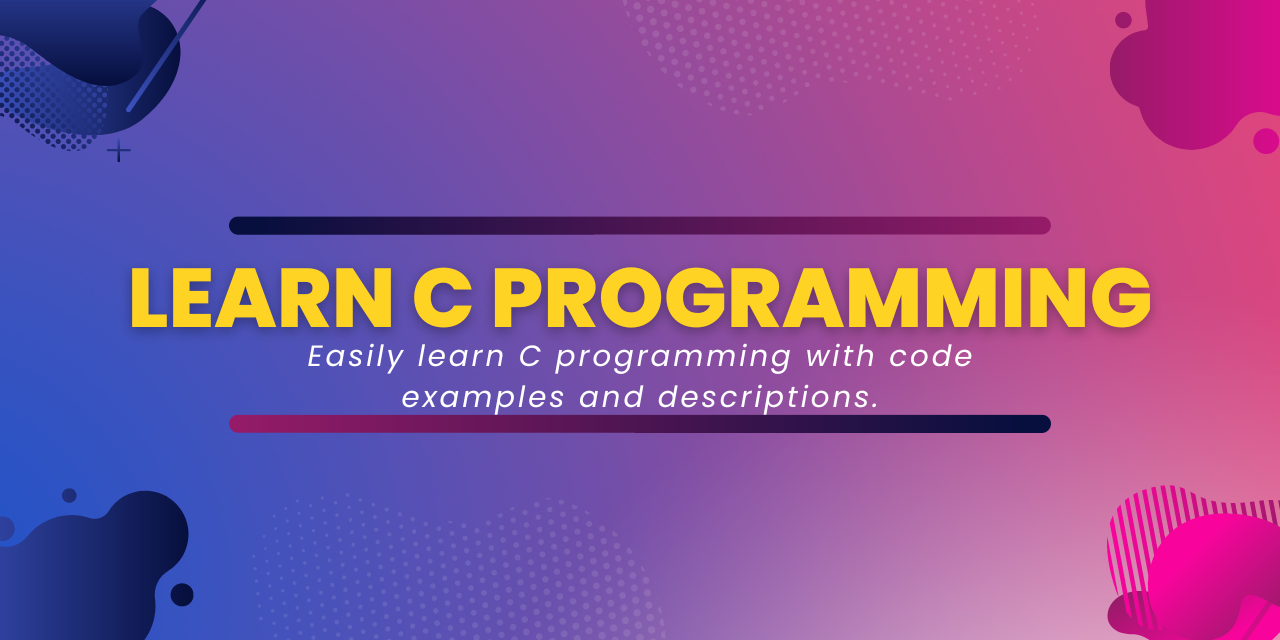 |
| 13 | + |
| 14 | +## 1. WAP to input 10 numbers and display the largest and smallest number. |
| 15 | + |
| 16 | +### Program |
| 17 | + |
| 18 | +```c |
| 19 | +//WAP to input 10 numbers and display the largest and smallest number. |
| 20 | + |
| 21 | +#include <stdio.h> |
| 22 | + |
| 23 | +int main(){ |
| 24 | + int a[10], largest, smallest; |
| 25 | + printf("Enter 10 numbers: "); |
| 26 | + for(int i=0; i< 10; i++){ |
| 27 | + scanf("%d", &a[i]); |
| 28 | + } |
| 29 | + largest = a[0]; |
| 30 | + smallest = a[1]; |
| 31 | + for(int i=0; i< 10; i++){ |
| 32 | + if(a[i] > largest){ |
| 33 | + largest = a[i]; |
| 34 | + } |
| 35 | + if(a[i] < smallest){ |
| 36 | + smallest = a[i]; |
| 37 | + } |
| 38 | + } |
| 39 | + printf("Largest: %d, smallest: %d\n",largest, smallest); |
| 40 | + return 0; |
| 41 | +} |
| 42 | +``` |
| 43 | + |
| 44 | +This program reads 10 numbers from the user and finds the largest and smallest |
| 45 | +number among them using two for loops. |
| 46 | + |
| 47 | +First, it declares an integer array of size 10 to store the numbers entered by |
| 48 | +the user. Then, it prompts the user to enter 10 numbers one by one using a for |
| 49 | +loop and scanf function. |
| 50 | + |
| 51 | +After that, it sets the initial values of largest and smallest as the first and |
| 52 | +second elements of the array, respectively. It then uses another for loop to |
| 53 | +compare all the elements of the array with the current value of largest and |
| 54 | +smallest. If any element of the array is larger than the current value of |
| 55 | +largest, the largest value is updated to that element. Similarly, if any element |
| 56 | +of the array is smaller than the current value of smallest, the smallest value |
| 57 | +is updated to that element. |
| 58 | + |
| 59 | +Finally, the program prints the largest and smallest numbers found during the |
| 60 | +comparison using printf function. |
| 61 | + |
| 62 | +## 2. WAP to read the age of 40 students and count the number of students aged between 15 and 22. |
| 63 | + |
| 64 | +### Program |
| 65 | + |
| 66 | +```c |
| 67 | +//WAP to read the age of 40 students and count the number of students aged between 15 and 22. |
| 68 | + |
| 69 | +#include <stdio.h> |
| 70 | +int main(){ |
| 71 | + int a[40], c=0; |
| 72 | + printf("Enter the age of students: "); |
| 73 | + for(int i=0; i<40; i++){ |
| 74 | + scanf("%d",&a[i]); |
| 75 | + if(a[i] >= 15 && a[i] <= 22) |
| 76 | + c++; |
| 77 | + } |
| 78 | + printf("Number of students between 15 to 22: %d\n", c); |
| 79 | +} |
| 80 | +``` |
| 81 | + |
| 82 | +This program is designed to read the age of 40 students and count the number of |
| 83 | +students aged between 15 and 22. |
| 84 | + |
| 85 | +Firstly, an integer array a of size 40 is declared to store the age of the 40 |
| 86 | +students. Then, the program prompts the user to enter the age of each student |
| 87 | +using a for loop that runs 40 times. Inside the loop, the scanf function is used |
| 88 | +to read each input age and store it in the corresponding element of the a array. |
| 89 | + |
| 90 | +After reading all the ages, another for loop is used to iterate through the a |
| 91 | +array and count the number of students whose age is between 15 and 22 |
| 92 | +(inclusive). If the age of the current student is within the given range, then |
| 93 | +the counter variable c is incremented. |
| 94 | + |
| 95 | +Finally, the program displays the number of students aged between 15 and 22 |
| 96 | +using the printf function. Note that the printf function does not need a format |
| 97 | +specifier for the integer c as it is a simple integer value. |
| 98 | + |
| 99 | +## 3. WAP to input several numbers and arrange them in ascending order. |
| 100 | + |
| 101 | +### Program |
| 102 | + |
| 103 | +```c |
| 104 | +//WAP to input several numbers and arrange them in ascending order. |
| 105 | + |
| 106 | +#include <stdio.h> |
| 107 | +int main(){ |
| 108 | + int a[10], temp; |
| 109 | + printf("Enter the elements of the array: "); |
| 110 | + for(int i=0; i<10; i++){ |
| 111 | + scanf("%d",&a[i]); |
| 112 | + } |
| 113 | + for(int i=0; i<10; i++){ |
| 114 | + for(int j=i+1; j<10; j++){ |
| 115 | + if(a[i] > a[j]){ |
| 116 | + temp = a[j]; |
| 117 | + a[j] = a[i]; |
| 118 | + a[i] = temp; |
| 119 | + } |
| 120 | + } |
| 121 | + } |
| 122 | + printf("Arranged numbers: \n"); |
| 123 | + for(int i=0; i<10; i++){ |
| 124 | + printf("%d\t", a[i]); |
| 125 | + } |
| 126 | + return 0; |
| 127 | +} |
| 128 | +``` |
| 129 | + |
| 130 | +This program takes input of 10 numbers and then arranges them in ascending order |
| 131 | +using the bubble sort algorithm. |
| 132 | + |
| 133 | +The input of the program is taken using the scanf() function and stored in an |
| 134 | +integer array called "a" with a size of 10. |
| 135 | + |
| 136 | +Then, two for loops are used for comparing and swapping the elements of the |
| 137 | +array in ascending order. The outer loop runs from 0 to 9, and the inner loop |
| 138 | +runs from i+1 to 9. This is because each element should be compared with all the |
| 139 | +remaining elements, which is done by the inner loop. |
| 140 | + |
| 141 | +If an element is found to be greater than the next element, then they are |
| 142 | +swapped. The swapping is done using a temporary variable called temp. |
| 143 | + |
| 144 | +Finally, the arranged numbers are printed using a for loop and printf() |
| 145 | +function. |
| 146 | + |
| 147 | +## 4. WAP to input a matrix of size rXc and transpose it. |
| 148 | + |
| 149 | +### Program |
| 150 | + |
| 151 | +```c |
| 152 | +//WAP to input a matrix of size rXc and transpose it. |
| 153 | +#include <stdio.h> |
| 154 | +int main(){ |
| 155 | + int a[3][3]; |
| 156 | + printf("Enter a matrix: "); |
| 157 | + for(int i=0; i<3; i++){ |
| 158 | + for(int j=0; j<3; j++){ |
| 159 | + scanf("%d",&a[i][j]); |
| 160 | + } |
| 161 | + } |
| 162 | + printf("Original matrix: \n"); |
| 163 | + for(int i=0; i<3; i++){ |
| 164 | + for(int j=0; j<3; j++){ |
| 165 | + printf("%d \t",a[i][j]); |
| 166 | + } |
| 167 | + printf("\n"); |
| 168 | + } |
| 169 | + printf("Transposed matrix: \n"); |
| 170 | + for(int i=0; i<3; i++){ |
| 171 | + for(int j=0; j<3; j++){ |
| 172 | + printf("%d \t",a[j][i]); |
| 173 | + } |
| 174 | + printf("\n"); |
| 175 | + } |
| 176 | +} |
| 177 | +``` |
| 178 | + |
| 179 | +This program takes a 3x3 matrix as input and then transposes it, which means it |
| 180 | +converts rows into columns and columns into rows. Here's a step-by-step |
| 181 | +explanation of the program: |
| 182 | + |
| 183 | +- The program declares a 3x3 integer array a to store the matrix. |
| 184 | +- The program then prompts the user to input the matrix by using two nested |
| 185 | + loops that iterate through each element of the matrix. |
| 186 | +- After the matrix is input, the program prints the original matrix by using two |
| 187 | + nested loops to iterate through each element and print it in a tabular format. |
| 188 | +- The program then prints the transposed matrix by using two nested loops to |
| 189 | + iterate through each element, but this time, it prints the elements of the |
| 190 | + array in transposed form. This is achieved by swapping the indices of rows and |
| 191 | + columns, so the row index becomes the column index and vice versa. |
| 192 | +- Finally, the program exits. |
| 193 | + |
| 194 | +Overall, this program demonstrates how to transpose a matrix in C using two |
| 195 | +nested loops to iterate through each element of the matrix. |
| 196 | + |
| 197 | +## 5. WAP to input two matrices of size 2X3 and display their sum. |
| 198 | + |
| 199 | +### Program |
| 200 | + |
| 201 | +```c |
| 202 | +//WAP to input two matrices of size 2X3 and display their sum. |
| 203 | + |
| 204 | +#include<stdio.h> |
| 205 | +int main(){ |
| 206 | + int a[2][3], b[2][3]; |
| 207 | + printf("Enter the first matrix:"); |
| 208 | + for(int i=0; i<2; i++){ |
| 209 | + for(int j=0; j<3; j++){ |
| 210 | + scanf("%d",&a[i][j]); |
| 211 | + } |
| 212 | + } |
| 213 | + printf("Enter the second matrix:"); |
| 214 | + for(int i=0; i<2; i++){ |
| 215 | + for(int j=0; j<3; j++){ |
| 216 | + scanf("%d",&b[i][j]); |
| 217 | + } |
| 218 | + } |
| 219 | + printf("Addition matrix: \n"); |
| 220 | + for(int i=0; i<2; i++){ |
| 221 | + for(int j=0; j<3; j++){ |
| 222 | + printf("%d\t",a[i][j] + b[i][j]); |
| 223 | + } |
| 224 | + printf("\n"); |
| 225 | + } |
| 226 | + return 0; |
| 227 | +} |
| 228 | +``` |
| 229 | + |
| 230 | +This program is designed to add two matrices of size 2x3. Here is an explanation |
| 231 | +of how the code works: |
| 232 | + |
| 233 | +- Two 2x3 matrices, a and b, are declared. |
| 234 | +- The user is prompted to enter the values for the first matrix, and these |
| 235 | + values are stored in a using a nested for loop. |
| 236 | +- The user is prompted to enter the values for the second matrix, and these |
| 237 | + values are stored in b using another nested for loop. |
| 238 | +- Another nested for loop is used to calculate the sum of the corresponding |
| 239 | + elements of a and b, and the result is printed as a new 2x3 matrix, which |
| 240 | + represents the addition of the two input matrices. |
| 241 | + |
| 242 | +The program works by adding the corresponding elements of a and b using a nested |
| 243 | +for loop to iterate over each element. The result is then printed as a new 2x3 |
| 244 | +matrix using another nested for loop to print each element in a row-column |
| 245 | +format. |
| 246 | + |
| 247 | +Note that in order for two matrices to be added, they must be of the same size |
| 248 | +and shape (i.e., have the same number of rows and columns). |
| 249 | + |
| 250 | +## 6. WAP to input custom values for two matrix along with their rows and columns and find the product of those two matrices. |
| 251 | + |
| 252 | +### Program |
| 253 | + |
| 254 | +```c |
| 255 | +// matrix multiplication |
| 256 | + |
| 257 | +#include <stdio.h> |
| 258 | +#include <stdlib.h> |
| 259 | +int mult_matrix(int, int, int, int); |
| 260 | + |
| 261 | +int main(int argc, char *argv[]) { |
| 262 | + int row1, column1, row2, column2; |
| 263 | + printf("================================\n"); |
| 264 | + printf("Enter number of rows and columns for the first matrix:\n"); |
| 265 | + scanf("%d%d", &row1, &column1); |
| 266 | + printf("================================\n"); |
| 267 | + printf("Enter number of rows and columns for the second matrix:\n"); |
| 268 | + scanf("%d%d", &row2, &column2); |
| 269 | + printf("================================\n"); |
| 270 | + // check to see if any of the rows and columns are negative |
| 271 | + if (((row1 * column1) < 1) && ((row2 * column2) < 1)) { |
| 272 | + printf("Enter non-negative value.\n"); |
| 273 | + exit(EXIT_FAILURE); |
| 274 | + } else { |
| 275 | + if (column1 != row2) { |
| 276 | + // check to see if the number of column of first matrix is equal to the |
| 277 | + // number of rows of second matrix; |
| 278 | + printf("Number of column of first matrix must be equal the row of second " |
| 279 | + "matrix.\n"); |
| 280 | + exit(EXIT_FAILURE); |
| 281 | + |
| 282 | + } else { |
| 283 | + // if all the conditions are true, then call the mult_matrix function |
| 284 | + mult_matrix(row1, column1, row2, column2); |
| 285 | + } |
| 286 | + } |
| 287 | + |
| 288 | + return 0; |
| 289 | +} |
| 290 | + |
| 291 | +int mult_matrix(int row1, int column1, int row2, int column2) { |
| 292 | + int arr1[row1][column1], arr2[row2][column2]; |
| 293 | + // take input for first matrix |
| 294 | + for (int i = 0; i < row1; i++) { |
| 295 | + for (int j = 0; j < column1; j++) { |
| 296 | + printf("For first matrix:\n"); |
| 297 | + printf("Enter element number [%d][%d]:\n", i + 1, j + 1); |
| 298 | + scanf("%d", &arr1[i][j]); |
| 299 | + } |
| 300 | + } |
| 301 | + |
| 302 | + printf("================================\n"); |
| 303 | + // take input for second matrix |
| 304 | + for (int i = 0; i < row2; i++) { |
| 305 | + for (int j = 0; j < column2; j++) { |
| 306 | + printf("For second matrix:\n"); |
| 307 | + printf("Enter element number [%d][%d]:\n", i + 1, j + 1); |
| 308 | + scanf("%d", &arr2[i][j]); |
| 309 | + } |
| 310 | + } |
| 311 | + printf("================================\n"); |
| 312 | + |
| 313 | + // multiplying the two matrixes |
| 314 | + int result[row1][column2]; |
| 315 | + for (int i = 0; i < row1; i++) { |
| 316 | + for (int j = 0; j < column2; j++) { |
| 317 | + result[i][j] = 0; |
| 318 | + for (int k = 0; k < column1; k++) { |
| 319 | + // either column1 or row2 can be used |
| 320 | + result[i][j] += arr1[i][k] * arr2[k][j]; |
| 321 | + } |
| 322 | + } |
| 323 | + } |
| 324 | + |
| 325 | + // muliplied matrix |
| 326 | + printf("Result of the product of those two matrixes\n"); |
| 327 | + for (int i = 0; i < row1; i++) { |
| 328 | + for (int j = 0; j < column2; j++) { |
| 329 | + printf("%d\t", result[i][j]); |
| 330 | + } |
| 331 | + printf("\n"); |
| 332 | + } |
| 333 | + |
| 334 | + return 0; |
| 335 | +} |
| 336 | +``` |
| 337 | +
|
| 338 | +- Takes input for the rows and columns from the user |
| 339 | +- Passes into a function with the name mult_matrix |
| 340 | +- Takes input for the values of those two matrix from the user |
| 341 | +- Multiplies the matrices |
| 342 | +
|
| 343 | +--- |
| 344 | +
|
| 345 | +#### LOGIC |
| 346 | +
|
| 347 | +The criteria for the multiplication of two matrices is that, |
| 348 | +
|
| 349 | +the number columns of the first matrix must be the same as the number of rows of |
| 350 | +second matrix. |
| 351 | +
|
| 352 | +example: matrices of order, 2x3 & 3x1 |
| 353 | +
|
| 354 | +are eligible for multiplication |
| 355 | +
|
| 356 | +--- |
| 357 | +
|
| 358 | +<!-- Add new question above this comment --> |
| 359 | +
|
| 360 | +## License |
| 361 | +
|
| 362 | +Released under [MIT](/LICENSE) by [@ComputeNepal](https://github.com/ComputeNepal). |
| 363 | +
|
| 364 | +[](https://computenepal.com) |
0 commit comments