-
Notifications
You must be signed in to change notification settings - Fork 0
Basics of Object Oriented Programming
Java and Kotlin are object oriented programming languages. If you want to make mods of your own it is crucial that you understand at least the basics concepts of object oriented programming. Here I will go over the core concepts with a focus on Kotlin. Things are a bit different in Java, but the core conceps are the same.
In Kotlin (and Java) basically everything is either an Object or a Class.
You can think of Classes as something similar to a blueprint, and Objects as the actual thing which is built from the blueprint. Like a blueprint Classes can in general not directly be interacted with. To make use of it you first have to create an instance of the Class called an Object.
A commonly used example is a Car, I will use that here as well. Classes can have properties and methods. Properties allow you to store data. In case of the car example this could the color. Methods contain the code that can be executed and basically allows you to do stuff. In the car example that could be something like fueling the car. In code a this can look like
class Car(val color: String, val driverSide: String) {
var fuelLevel = 0.5
fun fuelUp() {
fuelLevel = 1.0
}
}
You can then create an instance of the class by invoking the constructor. In Kotlin the main constructor is defined by the parentheses behind the class name.
val blueCar = Car("blue", "left")
val redCar = Car("red", "left")
Here the value blueCar
and redCar
hold a reference to the Object that is created by calling the constructor.
You can use that to invoke methods of the Object like fueling up the car.
blueCar.fuelUp()
This will set the fuleLevel
variable of the blueCar
Object to 1.0 while the redCar
remains unaffected.
For more information on this check out the Kotlin Docs.
An important aspect of Classes is that you can inherit from classes.
The Idea here is that you can have different classes which all share a certain set of properties and methods.
When we stick with our car example, this problem would arise when we now also wanted a class for a Motorbike.
We could then define a superclass Vehicle
that contains all the shared functionality
open class Vehicle(val color: String) {
var fuelLevel = 0.5
fun fuelUp() {
fuelLevel = 1.0
}
}
and inherit it from there. Note here the keyword open
. This is required to be able to inherit from the class.
Inheriting would then look like
class Car(color: String, val driverSide: String) : Vehicle(color) {}
This class would now behave the same as the Car class we defined earlier and you would still be able to invoke blueCar.fuelUp()
if we would have used this definition earlier.
For more information on this check out the Kotlin Docs.
Kotlin in a strictly typed language. This means that you always have to specify what kind of data your variables store, or your functions accept as inputs. In code you specify the type of your variables and function inputs like:
var name: Sting = ...
fun doSomething(parameter: Int) { ... }
The compiler helps you out here, and does that for you when the type is implicitly already given by the value you assign to a variable.
Since everything is an Object, so is the data you use and store. Therefore Types are Classes.
Get more info from the official documentation here and on the following pages.
I would also recommend checking out the page on Null Safety.
-
The official Kotlin Documentation. Especially check out the sections
Basics
andConcepts
. - A bit more in depth introduction that is not to lenghty.
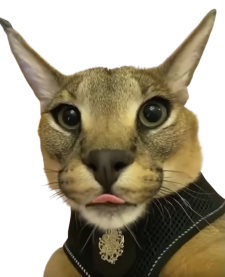