Commit b25f5e8
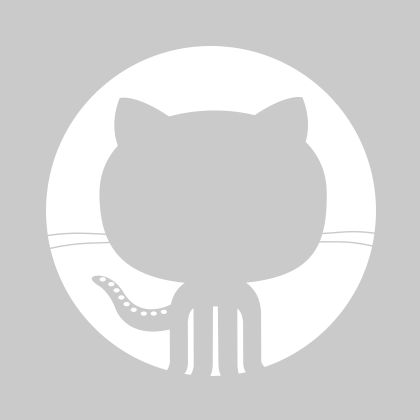
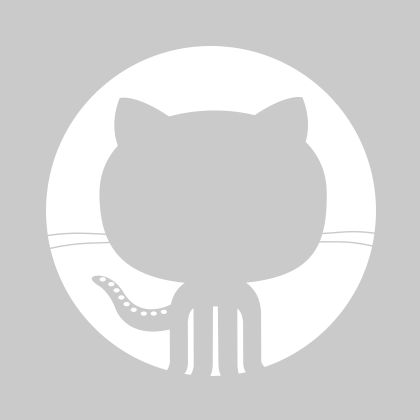
Samuel Ortiz
Samuel Ortiz
1 parent aaa36ec commit b25f5e8
3 files changed
+89
-83
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
63 | 63 |
| |
64 | 64 |
| |
65 | 65 |
| |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
| 147 | + | |
| 148 | + | |
| 149 | + |
-83
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
56 | 56 |
| |
57 | 57 |
| |
58 | 58 |
| |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
82 |
| - | |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
87 |
| - | |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
92 |
| - | |
93 |
| - | |
94 |
| - | |
95 |
| - | |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 |
| - | |
100 |
| - | |
101 |
| - | |
102 |
| - | |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
118 |
| - | |
119 |
| - | |
120 |
| - | |
121 |
| - | |
122 |
| - | |
123 |
| - | |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - |
0 commit comments