diff --git a/README.md b/README.md
index 90eaa4b..d87a7f3 100644
--- a/README.md
+++ b/README.md
@@ -3,8 +3,8 @@
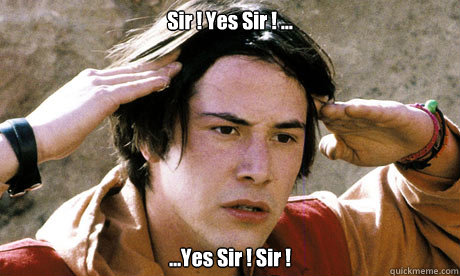
-This library provides an annotation, `@LogMe` that will log your functions in order to monitor their execution and duration.
-When a function is annotated, it will create a log before and after it's execution, showing it's execution time.
+This library provides an annotation, `@LogMe` that will log your functions in order to monitor their execution (with input parameters and results if those are available) and duration.
+When a function is annotated, it will create a log before and after it's execution, showing all the details.
For Example:
@@ -13,9 +13,11 @@ class Foo {
private val log = LoggerFactory.getLogger(this::class.java)
@LogMe
- fun foo(){
+ fun foo(name: String){
Thread.sleep(1000) // I.E.
- log.info("Hello World!")
+ val result = "Hello $name!"
+ log.info(result)
+ return result
}
}
```
@@ -23,9 +25,9 @@ class Foo {
When foo() is executed will result in:
``` shell
-23:29:26.969 [main] INFO com.mfalcier.yessir.Foo - com.mfalcier.yessir.Foo.foo has started its execution
-23:29:27.971 [main] INFO com.mfalcier.yessir.Foo - Hello World!
-23:29:27.972 [main] INFO com.mfalcier.yessir.Foo - com.mfalcier.yessir.Foo.foo has ended its execution after 1000ms
+23:29:26.969 [main] INFO com.mfalcier.yessir.Foo - [com.mfalcier.yessir.Foo.foo] has started its execution with parameters {name=Marco}
+23:29:27.971 [main] INFO com.mfalcier.yessir.Foo - Hello Marco!
+23:29:27.972 [main] INFO com.mfalcier.yessir.Foo - [com.mfalcier.yessir.Foo.foo] has ended its execution after 1000ms with result [Hello Marco!]
```
The `@LogMe` annotation can also be used on classes, in order to automatically log each of its method:
@@ -125,7 +127,7 @@ Maven:
com.github.mfalcier
YesSir
- 1.0.0
+ 1.1.0
...
diff --git a/pom.xml b/pom.xml
index 7d10c6d..01958a4 100644
--- a/pom.xml
+++ b/pom.xml
@@ -5,7 +5,7 @@
yessir
jar
YesSir
- 1.0.0
+ 1.1.0
1.8
@@ -121,6 +121,19 @@
+
+ org.apache.maven.plugins
+ maven-jar-plugin
+ 2.6
+
+
+
+ true
+ com.mfalcier.yessir.MainKt
+
+
+
+
diff --git a/src/main/kotlin/com/mfalcier/yessir/YesSir.kt b/src/main/kotlin/com/mfalcier/yessir/YesSir.kt
index 6603e16..50660c9 100644
--- a/src/main/kotlin/com/mfalcier/yessir/YesSir.kt
+++ b/src/main/kotlin/com/mfalcier/yessir/YesSir.kt
@@ -34,7 +34,7 @@ class YesSir {
val stopNanos = System.nanoTime()
val lengthMillis = TimeUnit.NANOSECONDS.toMillis(stopNanos - startNanos)
- afterExecution(joinPoint, lengthMillis)
+ afterExecution(joinPoint, lengthMillis, result)
return result
}
@@ -44,18 +44,33 @@ class YesSir {
val codeSignature = joinPoint.signature as CodeSignature
val cls = codeSignature.declaringType.name
val methodName = codeSignature.name
- val log = LoggerFactory.getLogger(cls)
+ val parameterNames = codeSignature.parameterNames
+ val parameterValues = joinPoint.args
+
+ val parameters = mutableMapOf()
+ parameterNames.forEachIndexed { index, name ->
+ parameters[name] = parameterValues[index]
+ }
- log.info("$cls.$methodName has started its execution")
+ val log = LoggerFactory.getLogger(cls)
+ if (parameters.isEmpty()) {
+ log.info("[$cls.$methodName] has started its execution")
+ } else {
+ log.info("[$cls.$methodName] has started its execution with parameters: $parameters")
+ }
}
- private fun afterExecution(joinPoint: JoinPoint, lengthMillis: Long) {
+ private fun afterExecution(joinPoint: JoinPoint, lengthMillis: Long, result: Any?) {
val signature = joinPoint.signature
val cls = signature.declaringType.name
val methodName = signature.name
val log = LoggerFactory.getLogger(cls)
- log.info("$cls.$methodName has ended its execution after ${lengthMillis}ms")
+ if (result == null) {
+ log.info("[$cls.$methodName] has ended its execution after ${lengthMillis}ms")
+ } else {
+ log.info("[$cls.$methodName] has ended its execution after ${lengthMillis}ms with result: [$result]")
+ }
}
}
\ No newline at end of file