-
Notifications
You must be signed in to change notification settings - Fork 143
New issue
Have a question about this project? # for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “#”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? # to your account
Performance improvements for build_histogram
and adjust_histogram
#855
Conversation
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Thank you very much for your wonderful insights Tim. I was actually intending to remove all of the exposure.jl
implementations from Images.jl
and replace them by reexporting from ImageContrastAdjustment.jl
. The build_histogram
code etc. lives there at the moment.
Perhaps we should open this pull-request over at ImageContrastAdjustment.jl
?
end | ||
|
||
function build_histogram(img::AbstractArray{T}) where T<:Color3{N0f8} | ||
build_histogram(mappedarray(Gray{N0f8}, img)) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I'm intrigued why the lazy mappedarray
is more performant than converting the entire image to Gray
using broadcast Gray.(img)
. I assumed that it is better to avoid any type of conversion inside hot loops. I would be grateful if you could take a moment to explain what is going on "under the hood" that makes this faster.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Since you only need each value once, there is no advantage in creating the array, so lazy mapping avoids the cost of allocation.
I feel our expectation on pushing Images.jl to v1.0 should be on API stabilization, code rearrangement, documentation, and versioning. Maintainable work on performance optimization and multi-threads requires more effort than we can pay in a short period (say, three months) and I One thing that might be irrelevant is, shall we consider to add more interchangeability to other libraries? Although I like the manner we reason in JuliaImages, PIL, scikit-images, and OpenCV are really good libraries and I think we should take advantage of them. Some folks around me are asking me to provide an OpenCV.jl wrapper even though I know nothing about C/C++ (e.g., opencv/opencv#16233.) JSoC 19' also listed this as a project option but nobody is really working on it so far. By saying this I think we need to standardize a way to "borrow" the functionality of other libraries for those we're not able to implement in a short time(lack of knowledge or time), with the artifacts feature in Julia 1.3 I think it's worth doing. |
I agree that across-the-board performance enhancement is something to work on later. But while we have a couple of folks writing benchmarks, I want them to know that we can fix some of the problems they are observing. A subset of methods in JuliaImages have been very carefully optimized, but it doesn't attract contributors if we're too slow on common tasks. And yes, I'm keenly interested in interop particularly with OpenCV. It's quite likely that the easiest way to systematically benchmark against OpenCV will be to have good wrappers. |
Will ImageContrastAdjustment move to JuliaImages? |
What's the process involved in moving the repo to JuliaImages? I believe it makes sense to move it, but I'd like to understand what moving it entails and what the implied social contract is once the repo is in JuliaImages. For example, at the moment the Documenter script that builds the documentation is linked with my Github account using my credentials. I wouldn't know how to configure the equivalent in JuliaImages. |
I've never done such work before, but as far as I understand, the porting strategy is
I think step 2, 3, and 4 can be done parallelly. Please correct me if there's anything I missed to consider.
These will be set under JuliaImages independently with the social account linked to JuliaImages, just consider them as two repositories that happens to have the same name. Only owners of JuliaImages or repositories under that have the permission to set up those secrets (for Documenter.jl). |
You should just be able to transfer the repo without duplicating it. Look
under the repo settings.
Steps 2 and 3 are still relevant.
|
In terms of social contract it's probably no different than for anything else that Images.jl depends on. Once Images.jl moved to JuliaImages, I don't think it makes sense to have Images.jl depend on anything "image-processing specific" that isn't hosted at JuliaImages. That's just insurance that important dependencies won't get abandoned by someone who doesn't merge PRs and doesn't add collaborators with sufficient permissions (not that I expect to have to worry about that in your case, but it's a good policy). If you have more specific questions about "social contract" let us know. |
Yes, I thought the previous URL will become invalid, but since it's actually get redirected to the new URL automatically, this isn't an issue. Transfering the whole repository is sufficient for step 1, thanks for the note @kmsquire
|
Let's move the porting discussions #841 |
I transferred my repository so its hosted over at the `JuliaImages` organsation (JuliaImages/Images.jl#855). This pull-request amends the repo URL so that it points to the new location.
I've transferred the |
Thanks @zygmuntszpak! Would you like to do the honors of rebasing Images.jl on ImageContrastAdjustment? I also think that would be a good time to deprecate |
I had actually started the process of updating the I am not entirely familiar with the deprecation strategy. Do I just reexport the functions in |
Yep, that's pretty much it. You can use something along the lines of Base.depwarn("`imhist` will be removed in a future release, please use `build_histogram` instead.", :imhist) |
I started out optimizing
imhist
(due to some benchmarks posted on the#image-processing
slack channel) but switched tobuild_histogram
as the more "sane" implementation.Setup:
Master:
This PR:
Comparison with
imhist
/histeq
:Not nearly as much an improvement for
adjust_histogram
. There is more that could be done but it would be more of an architecture change.These were inspired by comparison to PIL and OpenCV. With OpenCV, the naive approach fails for color images (https://stackoverflow.com/questions/15007304/histogram-equalization-not-working-on-color-image-opencv) and for PIL the quality is much worse than what we achieve:
Original:
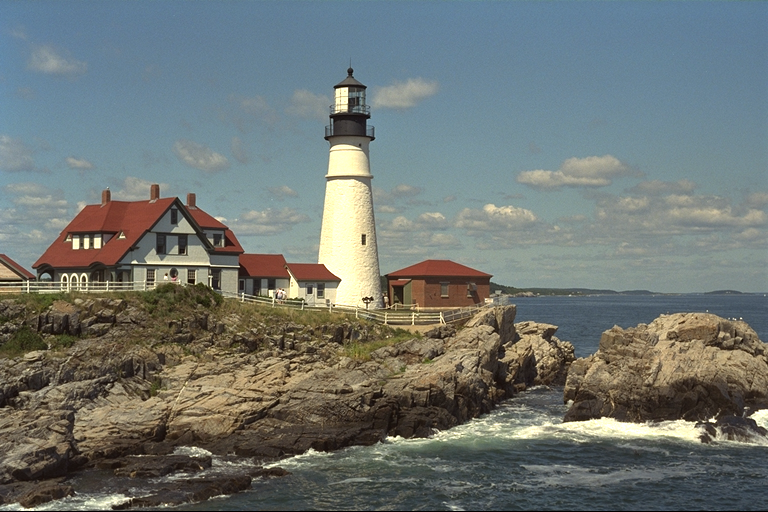
Julia's
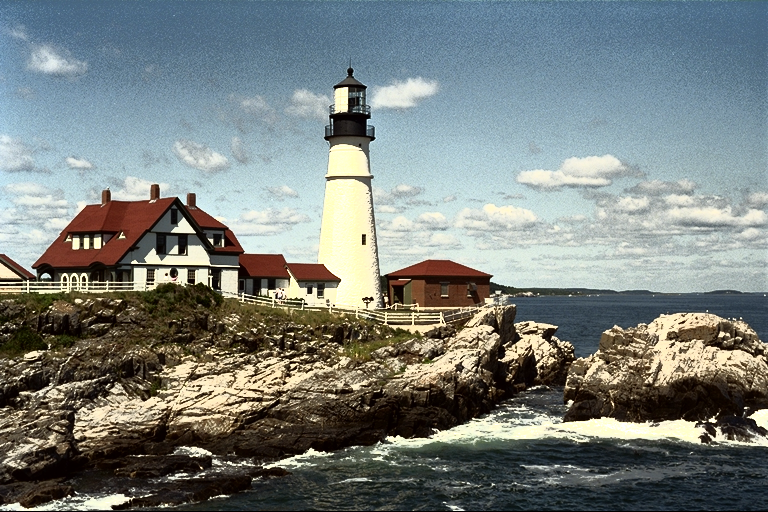
adjust_histogram
:PIL's
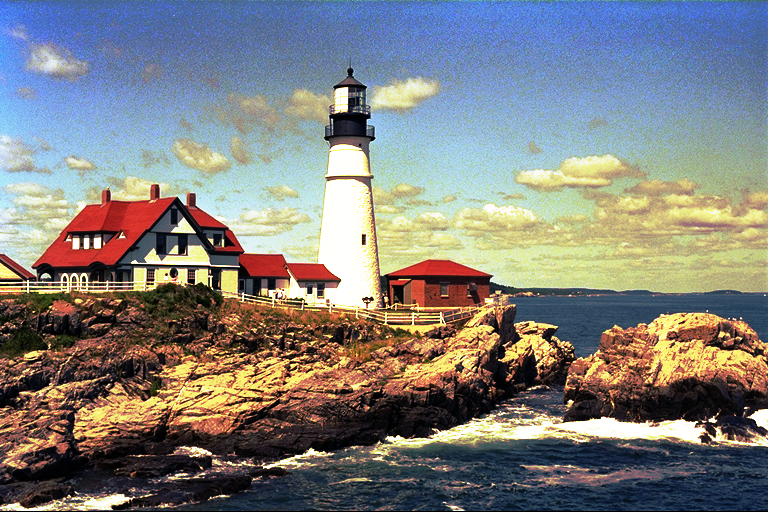
ImageOps.equalize
:You can see much more dramatic changes in hue with PIL.