-
Notifications
You must be signed in to change notification settings - Fork 25
Conversation
gl_Position = projection * view * position_world; | ||
gl_Position = projection * o_view_pos; | ||
// TODO calculate w/ normalmatrix (see o_normal links) | ||
o_view_normal = transpose(inverse(mat3(view))) * normal; |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Yeah we shouldn't calculate this in the shader ;)
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I added some code to generate a normalmatrix in cached_robj!()
Awesome :) On first sight, this looks pretty similar to how I'd have done it ;) |
src/rendering.jl
Outdated
# clear shouldn't be necessary. every pixel should be written to | ||
glClearColor(1, 0, 1, 1) | ||
glClear(GL_COLOR_BUFFER_BIT) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I made this clear color a jarring pink so I can see if it ever appears. Should be removed or set to white before merging. There's another one of these further down (using green)
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Pink becomes visible if bias < 0
.
// position in view space (as seen from camera) | ||
o_view_pos = view * position_world; | ||
// position in clip space (w/ depth) | ||
gl_Position = projection * o_view_pos; |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Do these require a v /= v.w
?
o_normal = normalmatrix * normal; | ||
// position in view space (as seen from camera) | ||
o_view_pos = view * position_world; | ||
// direction to light | ||
o_lightdir = normalize(lightposition - position_world.xyz); | ||
o_lightdir = normalize(view*vec4(lightposition, 1.0) - o_view_pos).xyz; | ||
// direction to camera | ||
o_camdir = normalize(eyeposition - position_world.xyz); | ||
// screen space coordinates of the vertex | ||
gl_Position = projection * view * position_world; | ||
o_camdir = normalize(view*vec4(eyeposition, 1.0) - o_view_pos).xyz; | ||
// position in clip space (w/ depth) | ||
gl_Position = projection * o_view_pos; |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Alternatively, we could also do
o_normal = normal_model_matrix * normal;
o_view_normal = normal_view_matrix * normal; // pass this to SSAO
o_lightdir = normalize(lightposition - position_world.xyz);
o_camdir = normalize(eyeposition - position_world.xyz);
o_view_pos = view * position_world; // pass this to SSAO
gl_Position = projection * o_view_pos;
where the normal matrices are transpose(inv(view)
and tranpose(inv(model))
. Not sure which is better...
using Colors, Makie
s1, radius = textslider(0.0f0:0.1f0:2f0, "Radius", start = 0.5f0)
s2, bias = textslider(0f0:0.005f0:0.1f0, "Bias", start = 0.025f0)
s3, blur = textslider(Int32(0):Int32(1):Int32(5), "Blur", start = Int32(2))
ssao_attrib = Attributes(radius=radius, bias=bias, blur=blur)
ps = rand(Point3f0, 100)
colors = rand(RGB, 100)
scene1 = meshscatter(ps, color=colors, ssao=true, SSAO=ssao_attrib)
scene2 = meshscatter(ps, color=colors, ssao=false) # default
hbox(vbox(s1, s2, s3), vbox(scene1, scene2)) Here's a little example for playing around with attributes. The left side uses SSAO, the right side doesn't. Depending on the colors the shine/glow issue is quite noticeable here, and by modifying |
Based on the learnopengl tutorial Simon posted in MakieOrg/Makie.jl#487
I believe SSAO is fully working at this point, but it's a very hacky implementation. I thought this would be a good point to get some feedback though, specifically on how connect this to GLMakie correctly.
Rough TODO
projection
in a less hacky waynumber of SSAO samples(requires shader recompilation - how?)maybe some controls for randomness of noise and samplesimplement deferred shading (i.e. move light calculations to postprocessing) (not doing this results in a weird glowing effect)(nvm, the glow is a result of blurring)allow SSAO to run w/o FXAArender_colorbuffer
Here are some pics:
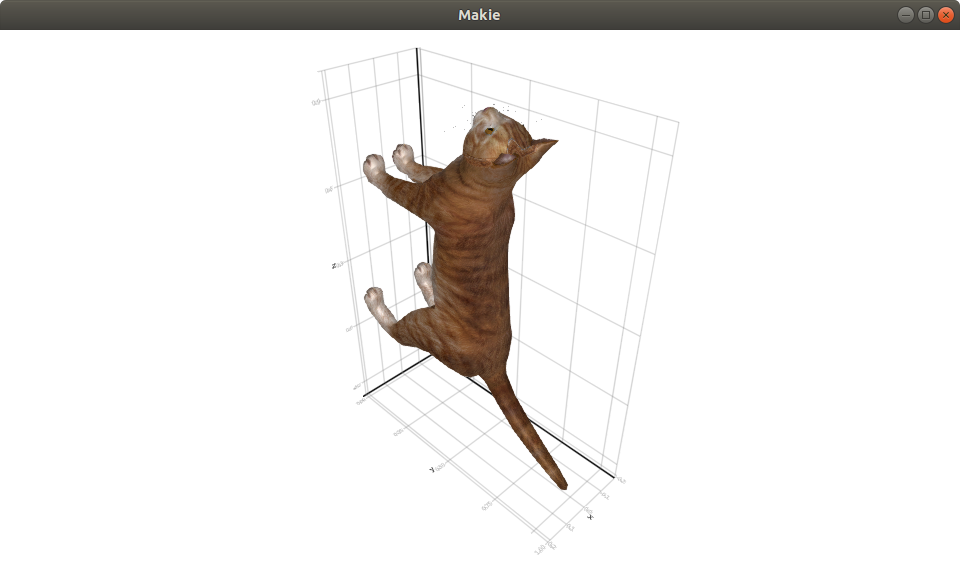
cat w/o SSAO:
cat w/ SSAO
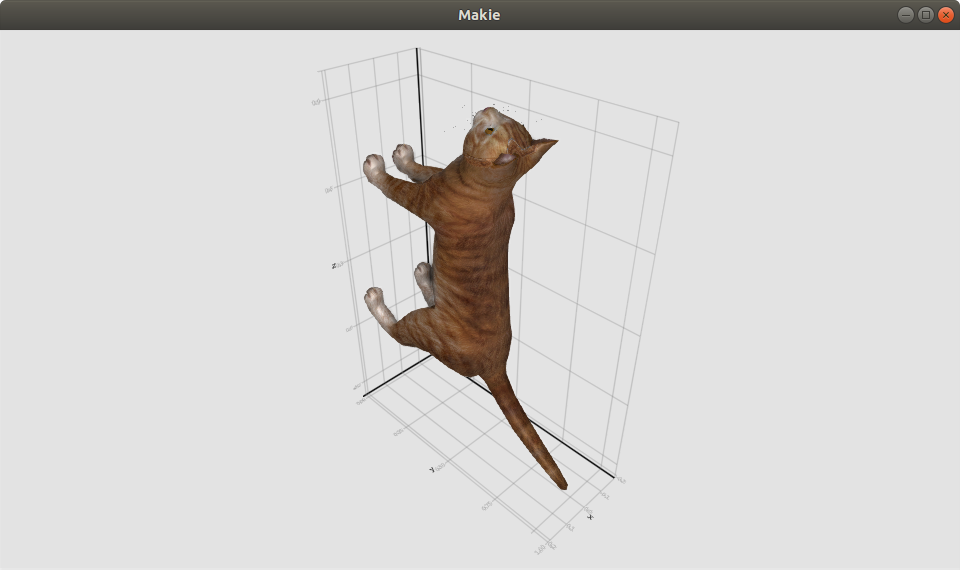
cat w/ only SSAO
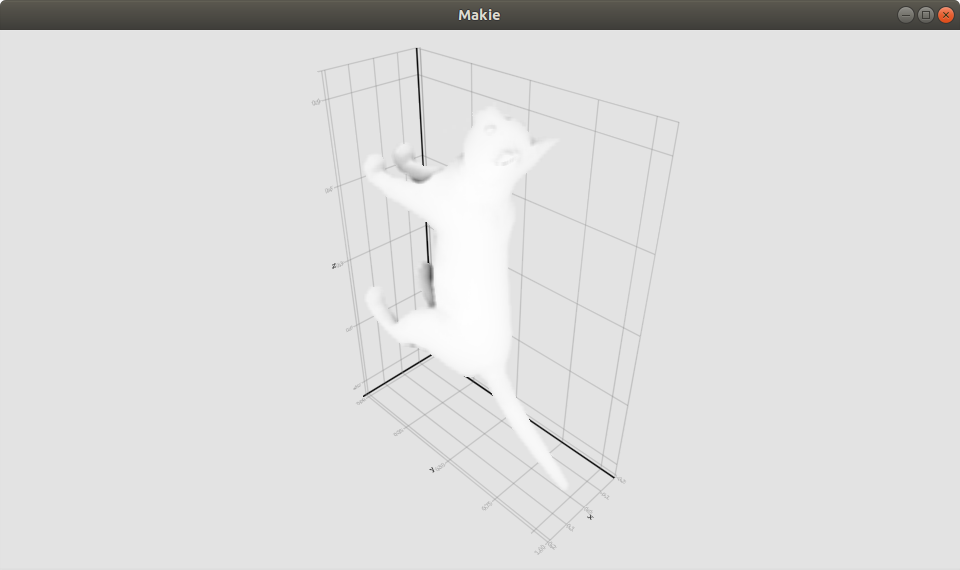